Lemmarx163
New member
Here is a tutorial to help implement Hiscore in projects. The following instructions have been done using the Maze tutorial.
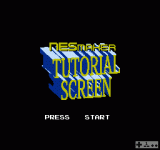
Prerequisites
- 1) Replace reset instructions in the program (JMP RESET)
- 2) Update the UpdateHudElement macro to allows multiples elements to be update in the same frame.
- 3) Add the UpdateHiScoreValue macro to handle the Hiscore
- Prerequisite 1 : Replace reset instructions in the program (JMP RESET)
To implement a Hiscore, a program should not restart using a hard reset because it will blank out all the memory and thus erase the Hiscore. The hardest part of this tutorial might be to implement a loop cycle in already existing programs.
There is 2 things to change to implement a loop cycle in a program.
1) Replace the RESET insctructions
2) Initialize variables to their initials values at the beginning of each new cycle (each new game)
---
1) Replace the RESET instructions
In the Maze Tutorial, there is 2 places where it uses JMP RESET.
1) When the player win (in the Input Editor - Press Start button of the Win Screen)
2) When there is no lives left (in the Handle Player Hurt)
Instead of resetting the game, the program will be change to cycle back to the Start Screen.
1) When the player win
- Go to the Input Editor
- Remove the mapping of the Press Start button of the Win Screen (simpleReset.asm)
- Add a new mapping for the Press Start button of the Win Screen to startGameWithNewContinuePoints.asm
- Open the Screen Info of the Win Screen (Overworld / Win Screen / Screen Info )
- Change the Warp out Screen X, Y to point to the Start screen X = 0, Y = 0
2) When there is no lives left
- Go to Project Setting / Script Settings
- Select Handle Player Hurt
- Click the Edit button to open the PlayerHurt_MazeBase.asm script
- Comment the line JMP RESET
- Add theses 2 lines of code
Now, the program should cycle to the Start Screen instead of resetting.
2) Initialize variables to their initials values at the beginning of each new cycle (each new game)
When the program resets (when it first start or reset), it clears the memory and initialize variables. The best moment to initialize variables of a new game is at the loading of the Start Screen. There is a script that run at the beginning of each screen load. This is where variables should be initialized.
- Go to Project Setting / Script Settings
- Select Extra Screen Load
- Click the Edit button to open the ExtraScreenLoadData_MazeBase.asm script
- Scroll to the bottom of the script and add theses lines of code
Now, the program should behave like it did before it cycles to the Start Screen instead of resetting.
- Prerequisite 2 : Update the UpdateHudElement macro
Follow instructions in this tutorial to allow the macro to update multiples elements of the hud in the same frame.
https://www.nesmakers.com/index.php?threads/update-multiple-hud-elements-simultaneously-4-5-9.8360/
- Prerequisite 3 : Add the UpdateHiScoreValue macro
Add the UpdateHiScoreValue code at the end of the UpdateHudElement script
If you know how to do it, it is highly recommended to create a new script for the UpdateHiScoreValue macro and add it to the macro list. It will help keep everything clean but it is out of the scope of this tutorial.
Now, everything is setup! Implementing the Hiscore system should be very easy for now on. Here is the 3 steps to do:
Steps :
- 1) Create an user variable to hold the Hiscore
- 2) Add the Hiscore to the Hud
- 3) Use the macro to update the Hiscore everywhere in the code where the score is update
- Step 1 : Create an user variable to hold the Hiscore
- Go to Project Setting / User Variables
- Add the myHiScore variables (the number of variables must fits the score variables, 6 in this case)
- Step 2 : Add the Hiscore to the Hud
- Go to HUD & Boxes / HUD Elements
- Add an Element for the Text Hi score
- Add an Element for the Number set to myHiScore and set the max value to 6 in this case
- Step 3 : Use the macro to update the Hiscore
In the Maze Tutorial, there is 2 places where the score increase.
1) In the tile prize script
2) In the pickup script
Use the UpdateHiScoreValue macro in each script to update the Hiscore
1) In the tile prize script
- Go to Project Setting / Script Settings
- Select Tile 05
- Click the Edit button to open the prize_MazeBase.asm script
- Insert theses lines just after UpdateHudElement #$03
2) In the pickup script
- Go to Project Setting / Script Settings
- Select Pickup Script
- Click the Edit button to open the templatePickupScript_MazeBase.asm script
- Insert theses lines just after UpdateHudElement #$03
That's it!
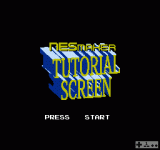
Prerequisites
- 1) Replace reset instructions in the program (JMP RESET)
- 2) Update the UpdateHudElement macro to allows multiples elements to be update in the same frame.
- 3) Add the UpdateHiScoreValue macro to handle the Hiscore
- Prerequisite 1 : Replace reset instructions in the program (JMP RESET)
To implement a Hiscore, a program should not restart using a hard reset because it will blank out all the memory and thus erase the Hiscore. The hardest part of this tutorial might be to implement a loop cycle in already existing programs.
There is 2 things to change to implement a loop cycle in a program.
1) Replace the RESET insctructions
2) Initialize variables to their initials values at the beginning of each new cycle (each new game)
---
1) Replace the RESET instructions
In the Maze Tutorial, there is 2 places where it uses JMP RESET.
1) When the player win (in the Input Editor - Press Start button of the Win Screen)
2) When there is no lives left (in the Handle Player Hurt)
Instead of resetting the game, the program will be change to cycle back to the Start Screen.
1) When the player win
- Go to the Input Editor
- Remove the mapping of the Press Start button of the Win Screen (simpleReset.asm)
- Add a new mapping for the Press Start button of the Win Screen to startGameWithNewContinuePoints.asm
- Open the Screen Info of the Win Screen (Overworld / Win Screen / Screen Info )
- Change the Warp out Screen X, Y to point to the Start screen X = 0, Y = 0
2) When there is no lives left
- Go to Project Setting / Script Settings
- Select Handle Player Hurt
- Click the Edit button to open the PlayerHurt_MazeBase.asm script
- Comment the line JMP RESET
- Add theses 2 lines of code
Code:
LDA #$00 ;Start screen YX coordinate
STA continueScreen
Now, the program should cycle to the Start Screen instead of resetting.
2) Initialize variables to their initials values at the beginning of each new cycle (each new game)
When the program resets (when it first start or reset), it clears the memory and initialize variables. The best moment to initialize variables of a new game is at the loading of the Start Screen. There is a script that run at the beginning of each screen load. This is where variables should be initialized.
- Go to Project Setting / Script Settings
- Select Extra Screen Load
- Click the Edit button to open the ExtraScreenLoadData_MazeBase.asm script
- Scroll to the bottom of the script and add theses lines of code
Code:
;Initialize game variables
LDA currentNametable ;Get the current screen number that is loading
CMP #$00 ;Compare the current screen loading number with the Start Screen number (00)
BNE +notStartScreen
;This code run only when we load the Start Screen
;Initialize variables here
LDA #$03
STA myLives
LDA #$00
STA myScore
STA myScore+1
STA myScore+2
STA myScore+3
STA myScore+4
STA myScore+5
;While we are here, we can initialize the player object initial states
StopMoving player1_object, #$FF, #$00
ChangeActionStep player1_object, #$00
ChangeFacingDirection player1_object, #FACE_DOWN
+notStartScreen
Now, the program should behave like it did before it cycles to the Start Screen instead of resetting.
- Prerequisite 2 : Update the UpdateHudElement macro
Follow instructions in this tutorial to allow the macro to update multiples elements of the hud in the same frame.
https://www.nesmakers.com/index.php?threads/update-multiple-hud-elements-simultaneously-4-5-9.8360/
- Prerequisite 3 : Add the UpdateHiScoreValue macro
Add the UpdateHiScoreValue code at the end of the UpdateHudElement script
Code:
MACRO UpdateHiScoreValue arg0, arg1, arg2
;arg0 = how many places this value has.
;arg1 = home variable for score
;arg2 = home variable for hi score
TYA
PHA
LDY arg0
DEY
-loop_evaluate_hiscore:
LDA arg1,y
CMP arg2,y
BCC +doneUpdateHiScore
BEQ +areEqual
;score is higher
JMP +doUpdateHiScore
+areEqual
DEY
BNE -loop_evaluate_hiscore
JMP +doneUpdateHiScore
+doUpdateHiScore
LDY arg0
DEY
-loop_update_hiscore:
LDA arg1,y
STA arg2,y
DEY
BNE -loop_update_hiscore
+doneUpdateHiScore
PLA
TAY
ENDM
If you know how to do it, it is highly recommended to create a new script for the UpdateHiScoreValue macro and add it to the macro list. It will help keep everything clean but it is out of the scope of this tutorial.
Now, everything is setup! Implementing the Hiscore system should be very easy for now on. Here is the 3 steps to do:
Steps :
- 1) Create an user variable to hold the Hiscore
- 2) Add the Hiscore to the Hud
- 3) Use the macro to update the Hiscore everywhere in the code where the score is update
- Step 1 : Create an user variable to hold the Hiscore
- Go to Project Setting / User Variables
- Add the myHiScore variables (the number of variables must fits the score variables, 6 in this case)
- Step 2 : Add the Hiscore to the Hud
- Go to HUD & Boxes / HUD Elements
- Add an Element for the Text Hi score
- Add an Element for the Number set to myHiScore and set the max value to 6 in this case
- Step 3 : Use the macro to update the Hiscore
In the Maze Tutorial, there is 2 places where the score increase.
1) In the tile prize script
2) In the pickup script
Use the UpdateHiScoreValue macro in each script to update the Hiscore
1) In the tile prize script
- Go to Project Setting / Script Settings
- Select Tile 05
- Click the Edit button to open the prize_MazeBase.asm script
- Insert theses lines just after UpdateHudElement #$03
Code:
;Update Hiscore
UpdateHiScoreValue #$06, myScore, myHiScore
UpdateHudElement #$05
2) In the pickup script
- Go to Project Setting / Script Settings
- Select Pickup Script
- Click the Edit button to open the templatePickupScript_MazeBase.asm script
- Insert theses lines just after UpdateHudElement #$03
Code:
;Update Hiscore
UpdateHiScoreValue #$06, myScore, myHiScore
UpdateHudElement #$05
That's it!