Lother
Member
Ok so the HandleHurtMonster.asm:
The Sprite Pre-Draw:
And the Use Sprite Based Weapon:
And the screenshot:
Hope it does help.
Code:
;;; what should we do with the monster?
;;; monster is loaded in x
LDA Object_vulnerability,x
AND #%00000100 ;; is it weapon immune?
BEQ notWeaponImmune
;PlaySound #SFX_MISS
JMP skipHurtingMonsterAndSound
notWeaponImmune:
LDA Object_status,x
AND #HURT_STATUS_MASK
BEQ dontskipHurtingMonster
JMP skipHurtingMonster
dontskipHurtingMonster:
LDA Object_status,x
ORA #%00000001
STA Object_status,x
LDA #HURT_TIMER
STA Object_timer_0,x
;;; assume idle is in step 0
ChangeObjectState #$00,#$02
;;;; unfortunately this recoil is backwards
LDA Object_status,x
AND #%00000100
BNE skipRecoilBecauseOnEdge
LDA Object_vulnerability,x
AND #%00001000
BNE skipRecoilBecauseOnEdge ;; skip recoil because bit is flipped to ignore recoil
LDA selfCenterX
STA recoil_otherX
LDA selfCenterY
STA recoil_otherY
LDA otherCenterX
STA recoil_selfX
LDA otherCenterY
STA recoil_selfY
JSR DetermineRecoilDirection
skipRecoilBecauseOnEdge:
LDA Object_health,x
SEC
SBC #$01
CMP #$01
BCC +
JMP notMonsterDeath
+
LDA Object_x_hi,x
STA temp
LDA Object_y_hi,x
STA temp1
DeactivateCurrentObject
CreateObject temp, temp1, #OBJ_MONSTER_DEATH, #$00, currentNametable ;Should create monster death
DeactivateCurrentObject
PlaySound #SND_SPLAT
;;;;;;;;;;;;;;;;;; ok, so now we also add points to score
;LDY Object_type,x
;LDA ObjectWorth,y
;STA temp
; AddValue #$03, GLOBAL_Player1_Score, temp
;arg0 = how many places this value has.
;arg1 = home variable
;arg2 = amount to add ... places?
;; and this should trip the update hud flag?
;;;;
TXA
STA tempy
AddValue #$08, myScore, #$01, #$00
;STA hudElementTilesToLoad
; LDA #$00
; STA hudElementTilesMax
; LDA DrawHudBytes
; ora #HUD_myScore
; STA DrawHudBytes
UpdateHud HUD_myScore
LDX tempy
JSR HandleDrops
JSR HandleToggleScrolling
CountObjects #$00001000, #$00
BEQ +
JMP ++
+
.include SCR_KILLED_LAST_MONSTER
++
JMP skipHurtingMonster
notMonsterDeath
STA Object_health,x
skipHurtingMonster:
;PlaySound #SFX_MONSTER_HURT
skipHurtingMonsterAndSound:
;;LDX tempx
;; what should we do with the projectile?
;;DeactivateCurrentObject
The Sprite Pre-Draw:
Code:
;; sprite pre-draw
;;; this will allow a user to draw sprites
;;; before object sprites are drawn.
;;; keep in mind, there are still only 64 sprites
;;; that can be drawn on a screen,
;;; and still only 8 per scan line!
;;; you can use DrawSprite macro directly using the following scheme:
;DrawSprite arg0, arg1, arg2, arg3, arg4
;arg0 = x
;arg1 = y
;arg2 = chr table value
;arg3 = attribute data
;arg3 = starting ram position
;; x and y are the direct positions on the screen in pixels.
;; chr table value is which sprite you'd like to draw from the ppu table.
;; attribute data is a binary number (starts with #%), and here are how the bits work:
;;; bit 7 - Flip sprite vertically
;;; bit 6 - Flip sprite horizontally
;;; bit 5 - priority (0 in front of background, 1 behind background)
;;; bit 4,3,2 - (null)
;;; bit 1,0 - subpalette used (00, 01, 10, 11)
;;; for starting ram position, use spriteOffset.
;; this is set to 0 just before entering this script (or 4 if sprite 0 hit was used).
;; ***remember to increase spriteOffset by 4 for every sprite that is drawn,
;; including after the last one drawn*****, so that the first object's sprite begins
;; in the next available spot.
;; I have created a macro function to handle updating to the next sprite. So, to update to the next sprite position,
;; all that you have to do is use the function UpdateSpritePointer
;;EXAMPLE:
; DrawSprite #$80, #$80, #$10, #%00000000, spriteOffset
; UpdateSpritePointer
;;;; DRAW SPRITE ZERO FOR SPRITE ZERO HIT
LDA gameState
CMP #GS_MainGame
BNE + ;dont draw sprite zero
;DrawSprite #$f8, #$1e, #$7F, #%00000000, spriteOffset
;248 30 127 bit 5 = priority
DrawSprite #SPRITE_ZERO_X, #SPRITE_ZERO_Y, #SPRITE_ZERO_INDEX, #%00100000, spriteOffset
UpdateSpritePointer
;dont draw sprite zero.
+
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
And the Use Sprite Based Weapon:
Code:
;;;Blank script
And the screenshot:
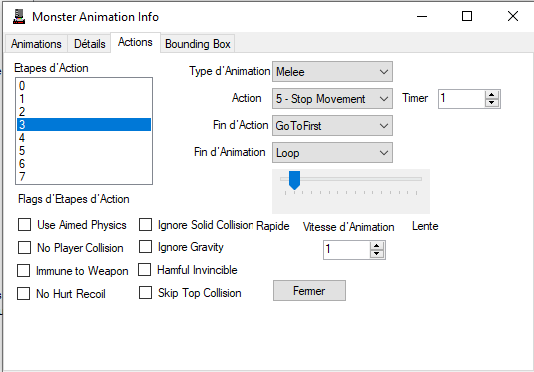
Hope it does help.